Revanth Vermareddy
Handling MongoDB GeoJson
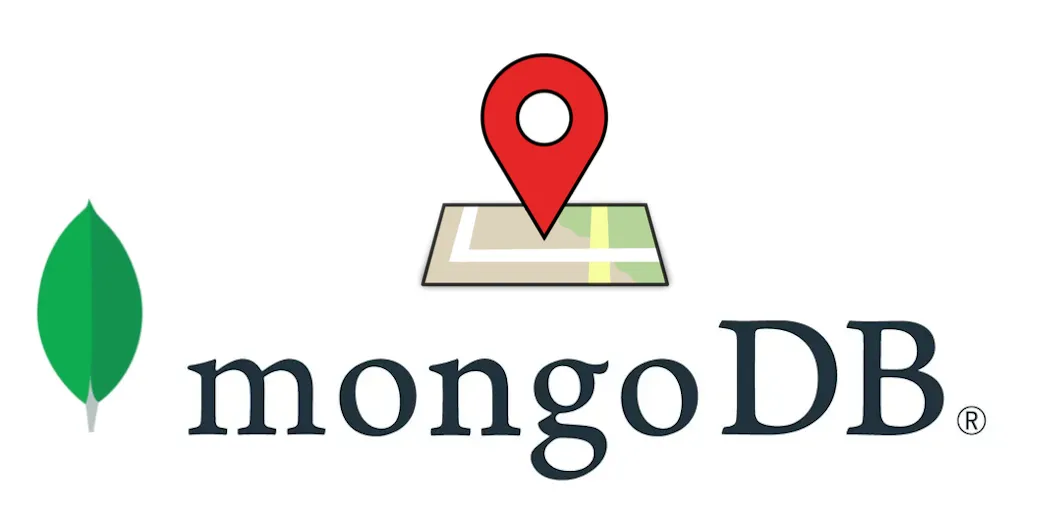
Whenever we have to store the location in MongoDb we usually encounter a confusion as to how to store the location co-ordinates
in the right way. Mongodb has a format called GeoJson
that handles the location in a more readable and intutive way.
This is how we store the legacy coordinate pairs in MongoDB:
"location": [-73.856077, 40.848447]
This is the format of storing the location as a GeoJson in mongodb:
"location": {
"type": "Point",
"coordinates": [-73.856077, 40.848447]
}
In mongodb there are 2 Geospatial Indexes possible:
2dsphere Indexes
- for spherical Earth models2d Indexes
- for flat Earth models
Spatial operators like $geoWithin
, $geoIntersects
, and $near
allow for precise filtering and retrieval of data based on
- proximity,
- containment, or
- intersection with our data.
read more: here
🔗Examples:
-
Example Finding the 5 closest points to me Lat, Lon
################################################################################ from pymongo import MongoClient # Connect to the MongoDB server client = MongoClient('mongodb://localhost:30000') # Access the database and collection db = client['johns_db'] collection = db['points_collection'] # User-defined latitude and longitude latitude = 30.2672 longitude = -97.7431 # Create the query to find the five nearest points query = { "location": { "$near": { "$geometry": { "type": "Point", "coordinates": [longitude, latitude] } } } } # Limit the results to the five nearest points projection = { "name": 1, "location": 1 } # Execute the query and find the five nearest points results = collection.find(query, projection).limit(5) # Print the results print("Five nearest points:") for point in results: print(f"Name: {point['name']}, Coordinates: {point['location']['coordinates']}") ################################################################################
-
Following the example using
$near
we’ll perform a$geoNearaggregation
(Note: This is too basic a use case for$geoNear
so consider aggregation pipelines when you want to string together transformation and query capabilities)################################################################################ from pymongo import MongoClient # Connect to the MongoDB server client = MongoClient('mongodb://localhost:30000') # Access the database and collection db = client['johns_db'] collection = db['points_collection'] # User-defined latitude and longitude latitude = 30.2672 longitude = -97.7431 # Create the aggregation pipeline pipeline = [ { "$geoNear": { "near": { "type": "Point", "coordinates": [user_longitude, user_latitude] }, "distanceField": "distance", "limit": 5, "spherical": True } } ] # Execute the aggregation pipeline results = collection.aggregate(pipeline) # Print the results print("Five nearest points:") for point in results: print(f"Name: {point['name']}, Coordinates: {point['location']['coordinates']}, Distance: {point['distance']}") ################################################################################